Beam-lib 2.15.1 |
#include <BString.h>
Public Member Functions | |
BString () | |
BString (const BString &string) | |
BString (const char *str) | |
BString (const char *str, unsigned int len) | |
BString (char ch) | |
BString (BInt v) | |
BString (BUInt v) | |
BString (BUInt64 v) | |
BString (double v) | |
~BString () | |
BString | copy () const |
Return an independant copy. More... | |
int | len () const |
Length of string. More... | |
const char * | retStr () const |
Ptr to char* representation. More... | |
char * | retStrDup () const |
Ptr to newly malloc'd char*. More... | |
int | retInt () const |
Return string as a int. More... | |
unsigned int | retUInt () const |
Return string as a int. More... | |
double | retDouble () const |
Return string as a double. More... | |
BFloat64 | retFloat64 () const |
Return string as a BFloat64. More... | |
int | compare (const BString &string) const |
Compare strings. More... | |
int | compareWild (const BString &string) const |
Compare string to string with wildcards. More... | |
int | compareWildExpression (const BString &string) const |
Compare string to space deliminated patterns. More... | |
int | compareRegex (const BString &pattern, int ignoreCase=0) const |
Compare strings. More... | |
BString & | truncate (int len) |
Truncate to length len. More... | |
BString & | pad (int len) |
Pad to length len. More... | |
void | clear () |
Clear the string. More... | |
BString & | toUpper () |
Convert to uppercase. More... | |
BString & | toLower () |
Convert to lowercase. More... | |
BString | lowerFirst () |
Return string with lowercase first character. More... | |
void | removeNL () |
Remove if present NL from last char. More... | |
BString | justify (int leftMargin, int width) |
Justify the string to the given width. More... | |
BString | fixedLen (int length, int rightJustify=0) |
return string formated to fixed length More... | |
BString | firstLine () |
Return first line. More... | |
BString | translateChar (char ch, BString replace=" ") |
Translate character converting them to the given string. More... | |
BString | reverse () const |
Reverse character order. More... | |
BString | subString (int start, int len) const |
Returns substring. More... | |
int | del (int start, int len) |
Delete substring. More... | |
int | insert (int start, BString str) |
Insert substring. More... | |
int | append (const BString &str) |
Append a string. More... | |
BString | add (const BString &str) const |
Add strings returning result. More... | |
BString & | printf (const char *fmt,...) |
Formated print into the string. More... | |
int | find (char ch) const |
Find ch in string searching forwards. More... | |
int | find (BString str) const |
Find string in string searching forwards. More... | |
int | findReverse (char ch) const |
Find ch in string searching backwards. More... | |
BString | csvEncode () const |
Encode a string for CSV. More... | |
BString & | csvDecode (const BString str) |
Decode a string from CSV. More... | |
BString | base64Encode () const |
Encode a string to base64. More... | |
BError | base64Decode (BString &str) const |
Decode a string from base64. More... | |
BList< BString > | getTokenList (BString separators) |
Break string into tokens. More... | |
BList< BString > | getTokenList (char separator) |
Break string into tokens. More... | |
BString | removeSeparators (BString separators) |
Remove any char from sepatators from string. More... | |
BString | pullToken (BString terminators) |
Pull token from start of string. More... | |
BString | pullSeparators (BString separators) |
Pull separators from start of string. More... | |
BString | pullWord () |
Pull a word out of the head of the string. More... | |
BString | pullLine () |
Pull a line out of the head of the string. More... | |
BList< BString > | split (char splitChar) |
Split string into an array based on the character separator. More... | |
BString | dirname () |
BString | basename () |
BString | extension () |
BUInt32 | hash () const |
char & | get (int pos) |
const char & | get (int pos) const |
BString & | operator= (const BString &string) |
char & | operator[] (int pos) |
int | operator== (const BString &s) const |
int | operator== (const char *s) const |
int | operator> (const BString &s) const |
int | operator> (const char *s) const |
int | operator< (const BString &s) const |
int | operator< (const char *s) const |
int | operator>= (const BString &s) const |
int | operator<= (const BString &s) const |
int | operator!= (const BString &s) const |
int | operator!= (const char *s) const |
BString | operator+ (const BString &s) const |
BString | operator+ (const char *s) const |
BString | operator+= (const BString &s) |
BString | operator+= (const char *s) |
BString | operator+ (char ch) const |
BString | operator+ (BInt i) const |
BString | operator+ (BUInt i) const |
BString | operator+ (BUInt64 i) const |
operator const char * () const | |
BString | field (int field) const |
char ** | fields () |
Static Public Member Functions | |
static BString | convert (char ch) |
Converts char to string. More... | |
static BString | convert (BInt value) |
Converts int to string. More... | |
static BString | convert (BUInt value) |
Converts uint to string. More... | |
static BString | convert (double value, int eFormat=0) |
Converts double to string. More... | |
static BString | convert (BUInt64 value) |
Converts long long to string. More... | |
static BString | convertHex (BInt value) |
Converts int to string as hex value. More... | |
static BString | convertHex (BUInt value) |
Converts uint to string as hex value. More... | |
Protected Attributes | |
BRefData * | ostr |
Private Member Functions | |
void | init (const char *str) |
int | inString (int pos) const |
int | isSpace (char ch) const |
Constructor & Destructor Documentation
◆ BString() [1/9]
BString::BString | ( | ) |
◆ BString() [2/9]
BString::BString | ( | const BString & | string | ) |
◆ BString() [3/9]
BString::BString | ( | const char * | str | ) |
◆ BString() [4/9]
BString::BString | ( | const char * | str, |
unsigned int | len | ||
) |
◆ BString() [5/9]
BString::BString | ( | char | ch | ) |
◆ BString() [6/9]
BString::BString | ( | BInt | v | ) |
◆ BString() [7/9]
BString::BString | ( | BUInt | v | ) |
◆ BString() [8/9]
BString::BString | ( | BUInt64 | v | ) |
◆ BString() [9/9]
BString::BString | ( | double | v | ) |
◆ ~BString()
BString::~BString | ( | ) |
Member Function Documentation
◆ add()
◆ append()
int BString::append | ( | const BString & | str | ) |
Append a string.
◆ base64Decode()
◆ base64Encode()
BString BString::base64Encode | ( | ) | const |
Encode a string to base64.
◆ basename()
BString BString::basename | ( | ) |
◆ clear()
void BString::clear | ( | ) |
Clear the string.
◆ compare()
int BString::compare | ( | const BString & | string | ) | const |
Compare strings.
◆ compareRegex()
int BString::compareRegex | ( | const BString & | pattern, |
int | ignoreCase = 0 | ||
) | const |
Compare strings.
◆ compareWild()
int BString::compareWild | ( | const BString & | string | ) | const |
Compare string to string with wildcards.
◆ compareWildExpression()
int BString::compareWildExpression | ( | const BString & | string | ) | const |
Compare string to space deliminated patterns.
◆ convert() [1/5]
| static |
Converts char to string.
◆ convert() [2/5]
◆ convert() [3/5]
◆ convert() [4/5]
| static |
Converts double to string.
◆ convert() [5/5]
◆ convertHex() [1/2]
◆ convertHex() [2/2]
◆ copy()
BString BString::copy | ( | ) | const |
Return an independant copy.
◆ csvDecode()
◆ csvEncode()
BString BString::csvEncode | ( | ) | const |
Encode a string for CSV.
◆ del()
int BString::del | ( | int | start, |
int | len | ||
) |
Delete substring.
◆ dirname()
BString BString::dirname | ( | ) |
◆ extension()
BString BString::extension | ( | ) |
◆ field()
BString BString::field | ( | int | field | ) | const |
◆ fields()
char ** BString::fields | ( | ) |
◆ find() [1/2]
int BString::find | ( | char | ch | ) | const |
Find ch in string searching forwards.
◆ find() [2/2]
int BString::find | ( | BString | str | ) | const |
Find string in string searching forwards.
◆ findReverse()
int BString::findReverse | ( | char | ch | ) | const |
Find ch in string searching backwards.
◆ firstLine()
BString BString::firstLine | ( | ) |
Return first line.
◆ fixedLen()
BString BString::fixedLen | ( | int | length, |
int | rightJustify = 0 | ||
) |
return string formated to fixed length
◆ get() [1/2]
char & BString::get | ( | int | pos | ) |
◆ get() [2/2]
const char & BString::get | ( | int | pos | ) | const |
◆ getTokenList() [1/2]
◆ getTokenList() [2/2]
◆ hash()
BUInt32 BString::hash | ( | ) | const |
◆ init()
| private |
◆ insert()
int BString::insert | ( | int | start, |
BString | str | ||
) |
Insert substring.
◆ inString()
| private |
◆ isSpace()
| private |
◆ justify()
BString BString::justify | ( | int | leftMargin, |
int | width | ||
) |
Justify the string to the given width.
◆ len()
int BString::len | ( | ) | const |
Length of string.
◆ lowerFirst()
BString BString::lowerFirst | ( | ) |
Return string with lowercase first character.
◆ operator const char *()
| inline |
◆ operator!=() [1/2]
| inline |
◆ operator!=() [2/2]
| inline |
◆ operator+() [1/6]
◆ operator+() [2/6]
| inline |
◆ operator+() [3/6]
| inline |
◆ operator+() [4/6]
◆ operator+() [5/6]
◆ operator+() [6/6]
◆ operator+=() [1/2]
◆ operator+=() [2/2]
| inline |
◆ operator<() [1/2]
| inline |
◆ operator<() [2/2]
| inline |
◆ operator<=()
| inline |
◆ operator=()
◆ operator==() [1/2]
| inline |
◆ operator==() [2/2]
| inline |
◆ operator>() [1/2]
| inline |
◆ operator>() [2/2]
| inline |
◆ operator>=()
| inline |
◆ operator[]()
char & BString::operator[] | ( | int | pos | ) |
◆ pad()
BString & BString::pad | ( | int | len | ) |
Pad to length len.
◆ printf()
BString & BString::printf | ( | const char * | fmt, |
... | |||
) |
Formated print into the string.
◆ pullLine()
BString BString::pullLine | ( | ) |
Pull a line out of the head of the string.
◆ pullSeparators()
◆ pullToken()
◆ pullWord()
BString BString::pullWord | ( | ) |
Pull a word out of the head of the string.
◆ removeNL()
void BString::removeNL | ( | ) |
Remove if present NL from last char.
◆ removeSeparators()
Remove any char from sepatators from string.
◆ retDouble()
double BString::retDouble | ( | ) | const |
Return string as a double.
◆ retFloat64()
BFloat64 BString::retFloat64 | ( | ) | const |
Return string as a BFloat64.
◆ retInt()
int BString::retInt | ( | ) | const |
Return string as a int.
◆ retStr()
const char * BString::retStr | ( | ) | const |
Ptr to char* representation.
◆ retStrDup()
char * BString::retStrDup | ( | ) | const |
Ptr to newly malloc'd char*.
◆ retUInt()
unsigned int BString::retUInt | ( | ) | const |
Return string as a int.
◆ reverse()
BString BString::reverse | ( | ) | const |
Reverse character order.
◆ split()
Split string into an array based on the character separator.
◆ subString()
BString BString::subString | ( | int | start, |
int | len | ||
) | const |
Returns substring.
◆ toLower()
BString & BString::toLower | ( | ) |
Convert to lowercase.
◆ toUpper()
BString & BString::toUpper | ( | ) |
Convert to uppercase.
◆ translateChar()
Translate character converting them to the given string.
◆ truncate()
BString & BString::truncate | ( | int | len | ) |
Truncate to length len.
Member Data Documentation
◆ ostr
| protected |
The documentation for this class was generated from the following files:
Generated by
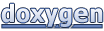