Beam-lib 2.15.1 |
Template based list class. More...
#include <BList.h>

Classes | |
class | Node |
Public Types | |
typedef int(* | SortFunc) (T &a, T &b) |
Prototype for sorting function. More... | |
Public Member Functions | |
BList () | |
BList (const BList< T > &l) | |
virtual | ~BList () |
void | start (BIter &i) const |
Iterator to start of list. More... | |
BIter | begin () const |
Iterator for start of list. More... | |
BIter | end () const |
Iterator for end of list. More... | |
BIter | end (BIter &i) const |
Iterator for end of list. More... | |
void | next (BIter &i) const |
Iterator for next item in list. More... | |
void | prev (BIter &i) |
Iterator for previous item in list. More... | |
BIter | goTo (int pos) const |
Iterator for pos item in list. More... | |
int | position (BIter i) |
Postition in list item with iterator i. More... | |
unsigned int | number () const |
Number of items in list. More... | |
unsigned int | size () const |
Number of items in list. More... | |
int | isEnd (BIter &i) const |
True if iterator refers to last item. More... | |
T & | front () |
Get first item in list. More... | |
T & | rear () |
Get last item in list. More... | |
T & | get (BIter i) |
Get item specified by iterator in list. More... | |
const T & | get (BIter i) const |
Get item specified by iterator in list. More... | |
void | append (const T &item) |
Append item to list. More... | |
virtual void | insert (BIter &i, const T &item) |
Insert item before item. More... | |
void | insertAfter (BIter &i, const T &item) |
Insert item after item. More... | |
virtual void | clear () |
Clear the list. More... | |
virtual void | del (BIter &i) |
Delete specified item. More... | |
void | deleteLast () |
Delete last item. More... | |
void | deleteFirst () |
Delete fisrt item. More... | |
void | push (const T &i) |
Push item onto list. More... | |
T | pop () |
Pop item from list deleteing item. More... | |
void | queueAdd (const T &i) |
Add item to end of list. More... | |
T | queueGet () |
Get item from front of list deleteing item. More... | |
void | append (const BList< T > &l) |
Append list to list. More... | |
int | has (const T &i) const |
Checks if the item is in the list. More... | |
void | swap (BIter i1, BIter i2) |
Swap two items in list. More... | |
void | sort () |
Sort list based on get(i) values. More... | |
void | sort (SortFunc func) |
Sort list based on Sort func. More... | |
BList< T > & | operator= (const BList< T > &l) |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
T & | operator[] (BIter i) |
const T & | operator[] (const BIter &i) const |
BList< T > | operator+ (const BList< T > &l) const |
Protected Member Functions | |
virtual Node * | nodeGet (BIter i) |
virtual const Node * | nodeGet (BIter i) const |
virtual Node * | nodeCreate (const T &item) |
Protected Attributes | |
Node * | onodes |
unsigned int | olength |
Private Member Functions | |
virtual Node * | nodeCreate () |
Detailed Description
template<class T>
class BList< T >
Template based list class.
Member Typedef Documentation
◆ SortFunc
typedef int(* BList< T >::SortFunc) (T &a, T &b) |
Prototype for sorting function.
Constructor & Destructor Documentation
◆ BList() [1/2]
◆ BList() [2/2]
◆ ~BList()
Member Function Documentation
◆ append() [1/2]
void BList< T >::append | ( | const T & | item | ) |
Append item to list.
◆ append() [2/2]
◆ begin()
◆ clear()
| virtual |
Clear the list.
Reimplemented in BEntryFile, BDir, BEntryList, BDict< Type >, BQueue< T >, and BQueue< BoapMcPacket >.
◆ del()
Delete specified item.
Reimplemented in BEntryList, and BDict< Type >.
◆ deleteFirst()
void BList< T >::deleteFirst | ( | ) |
Delete fisrt item.
◆ deleteLast()
void BList< T >::deleteLast | ( | ) |
Delete last item.
◆ end() [1/2]
◆ end() [2/2]
◆ front()
T & BList< T >::front | ( | ) |
Get first item in list.
◆ get() [1/2]
◆ get() [2/2]
Get item specified by iterator in list.
◆ goTo()
◆ has()
int BList< T >::has | ( | const T & | i | ) | const |
Checks if the item is in the list.
◆ insert()
Insert item before item.
Reimplemented in BEntryList, and BDict< Type >.
◆ insertAfter()
Insert item after item.
◆ isEnd()
◆ next()
◆ nodeCreate() [1/2]
◆ nodeCreate() [2/2]
◆ nodeGet() [1/2]
◆ nodeGet() [2/2]
◆ number()
unsigned int BList< T >::number | ( | ) | const |
Number of items in list.
◆ operator+()
◆ operator=()
◆ operator[]() [1/4]
T & BList< T >::operator[] | ( | int | i | ) |
◆ operator[]() [2/4]
const T & BList< T >::operator[] | ( | int | i | ) | const |
◆ operator[]() [3/4]
◆ operator[]() [4/4]
◆ pop()
T BList< T >::pop | ( | ) |
Pop item from list deleteing item.
◆ position()
◆ prev()
◆ push()
void BList< T >::push | ( | const T & | i | ) |
Push item onto list.
◆ queueAdd()
void BList< T >::queueAdd | ( | const T & | i | ) |
Add item to end of list.
◆ queueGet()
T BList< T >::queueGet | ( | ) |
Get item from front of list deleteing item.
◆ rear()
T & BList< T >::rear | ( | ) |
Get last item in list.
◆ size()
unsigned int BList< T >::size | ( | ) | const |
Number of items in list.
◆ sort() [1/2]
void BList< T >::sort | ( | ) |
Sort list based on get(i) values.
◆ sort() [2/2]
◆ start()
◆ swap()
Member Data Documentation
◆ olength
| protected |
◆ onodes
The documentation for this class was generated from the following files:
Generated by
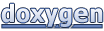