Beam-lib 2.15.1 |
#include <BFifo.h>
Public Member Functions | |
BFifo (BUInt size) | |
~BFifo () | |
void | clear () |
BUInt | size () |
Returns fifo size. More... | |
BError | resize (BUInt size) |
Resize FIFO, clears it as well. More... | |
BError | rebase () |
Rebases fifo so read pointer is at zero moving memory as needed. More... | |
BUInt | writeAvailable () |
How many items that can be written. More... | |
BUInt | writeAvailableChunk () |
How many items that can be written in a chunk. More... | |
BError | write (const Type v) |
Write a single item. More... | |
BError | write (const Type *data, BUInt num) |
Write a set of items. Can only write a maximum of writeAvailableChunk() to save going beyond end of FIFO buffer. More... | |
Type * | writeData () |
Returns a pointer to the data. More... | |
Type * | writeData (BUInt &num) |
Returns a pointer to the data and how many can be written in a chunk. More... | |
void | writeDone (BUInt num) |
Indicates when write is complete. More... | |
void | writeBackup (BUInt num) |
Backup, remove num items at end of fifo. Careful, make sure read is not already happening. More... | |
BUInt | readAvailable () |
How many items are available to read. More... | |
BUInt | readAvailableChunk () |
How many items are available to read in a chunk. More... | |
Type | read () |
Read one item. More... | |
BError | read (Type *data, BUInt num) |
Read a set of items. More... | |
Type * | readData () |
Returns a pointer to the data. More... | |
Type * | readData (BUInt &num) |
Returns a pointer to the data and how many can be read in a chunk. More... | |
void | readDone (BUInt num) |
Type | readPos (BUInt pos) |
Read item at given offset from current read position. More... | |
void | writePos (BUInt pos, const Type &v) |
Write item at given offset from current read position. More... | |
Type & | operator[] (int pos) |
Direct access to read samples in buffer. More... | |
Protected Attributes | |
BUInt | osize |
The size of the FIFO. More... | |
Type * | odata |
FIFO memory buffer. More... | |
volatile BUInt | owritePos |
The write pointer. More... | |
volatile BUInt | oreadPos |
The read pointer. More... | |
Constructor & Destructor Documentation
◆ BFifo()
◆ ~BFifo()
Member Function Documentation
◆ clear()
void BFifo< Type >::clear | ( | ) |
◆ operator[]()
Type& BFifo< Type >::operator[] | ( | int | pos | ) |
Direct access to read samples in buffer.
◆ read() [1/2]
Type BFifo< Type >::read | ( | ) |
Read one item.
◆ read() [2/2]
◆ readAvailable()
◆ readAvailableChunk()
How many items are available to read in a chunk.
◆ readData() [1/2]
Type* BFifo< Type >::readData | ( | ) |
Returns a pointer to the data.
◆ readData() [2/2]
Returns a pointer to the data and how many can be read in a chunk.
◆ readDone()
◆ readPos()
Read item at given offset from current read position.
◆ rebase()
Rebases fifo so read pointer is at zero moving memory as needed.
◆ resize()
◆ size()
◆ write() [1/2]
◆ write() [2/2]
Write a set of items. Can only write a maximum of writeAvailableChunk() to save going beyond end of FIFO buffer.
◆ writeAvailable()
◆ writeAvailableChunk()
How many items that can be written in a chunk.
◆ writeBackup()
Backup, remove num items at end of fifo. Careful, make sure read is not already happening.
◆ writeData() [1/2]
Type* BFifo< Type >::writeData | ( | ) |
Returns a pointer to the data.
◆ writeData() [2/2]
Returns a pointer to the data and how many can be written in a chunk.
◆ writeDone()
◆ writePos()
Write item at given offset from current read position.
Member Data Documentation
◆ odata
| protected |
FIFO memory buffer.
◆ oreadPos
◆ osize
◆ owritePos
The documentation for this class was generated from the following file:
Generated by
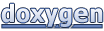