Beam-lib 2.15.1 |
BEntryList Class Reference
List of Entries. Where an entry is a name value pair. More...
#include <BEntry.h>
Inheritance diagram for BEntryList:
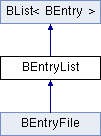
Public Member Functions | |
BEntryList () | |
int | isSet (BString name) |
1 if name is in list and value is set More... | |
BEntry * | find (BString name) |
Returns entry if name is found otherwise NULL. More... | |
BString | findValue (BString name) |
Returns value of name. Returns "" if name not found. More... | |
int | setValue (BString name, BString value) |
Set the value of name. Returns 0 if name not found. More... | |
int | setValueRaw (BString name, BString value) |
Raw setting of value without looking up existing entry. More... | |
void | deleteEntry (BString name) |
Deletes the entry. More... | |
void | print () |
Print list. More... | |
BString | getString () |
Return list as string. Each Entry padded and on a new line. More... | |
void | insert (BIter &i, const BEntry &item) |
Insert item before item. More... | |
void | del (BIter &i) |
Delete specified item. More... | |
void | clear () |
Clear the list. More... | |
BEntryList & | operator= (const BEntryList &l) |
![]() | |
BList () | |
BList (const BList< BEntry > &l) | |
virtual | ~BList () |
void | start (BIter &i) const |
Iterator to start of list. More... | |
BIter | begin () const |
Iterator for start of list. More... | |
BIter | end () const |
Iterator for end of list. More... | |
BIter | end (BIter &i) const |
Iterator for end of list. More... | |
void | next (BIter &i) const |
Iterator for next item in list. More... | |
void | prev (BIter &i) |
Iterator for previous item in list. More... | |
BIter | goTo (int pos) const |
Iterator for pos item in list. More... | |
int | position (BIter i) |
Postition in list item with iterator i. More... | |
unsigned int | number () const |
Number of items in list. More... | |
unsigned int | size () const |
Number of items in list. More... | |
int | isEnd (BIter &i) const |
True if iterator refers to last item. More... | |
BEntry & | front () |
Get first item in list. More... | |
BEntry & | rear () |
Get last item in list. More... | |
BEntry & | get (BIter i) |
Get item specified by iterator in list. More... | |
const BEntry & | get (BIter i) const |
Get item specified by iterator in list. More... | |
void | append (const BEntry &item) |
Append item to list. More... | |
void | append (const BList< BEntry > &l) |
Append list to list. More... | |
void | insertAfter (BIter &i, const BEntry &item) |
Insert item after item. More... | |
void | deleteLast () |
Delete last item. More... | |
void | deleteFirst () |
Delete fisrt item. More... | |
void | push (const BEntry &i) |
Push item onto list. More... | |
BEntry | pop () |
Pop item from list deleteing item. More... | |
void | queueAdd (const BEntry &i) |
Add item to end of list. More... | |
BEntry | queueGet () |
Get item from front of list deleteing item. More... | |
int | has (const BEntry &i) const |
Checks if the item is in the list. More... | |
void | swap (BIter i1, BIter i2) |
Swap two items in list. More... | |
void | sort () |
Sort list based on get(i) values. More... | |
void | sort (SortFunc func) |
Sort list based on Sort func. More... | |
BList< BEntry > & | operator= (const BList< BEntry > &l) |
BEntry & | operator[] (int i) |
const BEntry & | operator[] (int i) const |
BEntry & | operator[] (BIter i) |
const BEntry & | operator[] (const BIter &i) const |
BList< BEntry > | operator+ (const BList< BEntry > &l) const |
Private Attributes | |
BIter | olastPos |
Additional Inherited Members | |
![]() | |
typedef int(* | SortFunc) (BEntry &a, BEntry &b) |
Prototype for sorting function. More... | |
![]() | |
virtual Node * | nodeGet (BIter i) |
virtual const Node * | nodeGet (BIter i) const |
virtual Node * | nodeCreate (const BEntry &item) |
![]() | |
Node * | onodes |
unsigned int | olength |
Detailed Description
List of Entries. Where an entry is a name value pair.
Constructor & Destructor Documentation
◆ BEntryList()
BEntryList::BEntryList | ( | ) |
Member Function Documentation
◆ clear()
| virtual |
◆ del()
| virtual |
Delete specified item.
Reimplemented from BList< BEntry >.
◆ deleteEntry()
void BEntryList::deleteEntry | ( | BString | name | ) |
Deletes the entry.
◆ find()
◆ findValue()
◆ getString()
BString BEntryList::getString | ( | ) |
Return list as string. Each Entry padded and on a new line.
◆ insert()
Insert item before item.
Reimplemented from BList< BEntry >.
◆ isSet()
int BEntryList::isSet | ( | BString | name | ) |
1 if name is in list and value is set
◆ operator=()
BEntryList & BEntryList::operator= | ( | const BEntryList & | l | ) |
◆ print()
void BEntryList::print | ( | ) |
Print list.
◆ setValue()
Set the value of name. Returns 0 if name not found.
◆ setValueRaw()
Raw setting of value without looking up existing entry.
Member Data Documentation
◆ olastPos
| private |
The documentation for this class was generated from the following files:
Generated by
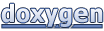