Beam-lib 2.15.1 |
Public Types | Public Member Functions | Private Member Functions | Private Attributes | List of all members
BDict< Type > Class Template Reference
#include <BDict.h>
Inheritance diagram for BDict< Type >:
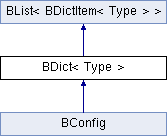
Public Types | |
typedef BIter | iterator |
![]() | |
typedef int(* | SortFunc) (BDictItem< Type > &a, BDictItem< Type > &b) |
Prototype for sorting function. More... | |
Public Member Functions | |
BDict (int hashSize=100) | |
BDict (const BDict< Type > &dict) | |
int | hasKey (const BString &k) const |
BString | key (const BIter &i) const |
void | clear () |
Clear the list. More... | |
void | insert (BIter &i, const BDictItem< Type > &item) |
Insert item before item. More... | |
void | append (const BDictItem< Type > &item) |
void | append (const BDict< Type > &dict) |
void | del (const BString &k) |
void | del (BIter &i) |
Delete specified item. More... | |
BIter | find (const BString &k) const |
Type & | operator[] (const BString &i) |
const Type & | operator[] (const BString &i) const |
Type & | operator[] (const BIter &i) |
const Type & | operator[] (const BIter &i) const |
BDict< Type > | operator+ (const BDict< Type > &dict) const |
BDict< Type > & | operator= (const BDict< Type > &dict) |
void | hashPrint () |
![]() | |
BList () | |
BList (const BList< BDictItem< Type > > &l) | |
virtual | ~BList () |
void | start (BIter &i) const |
Iterator to start of list. More... | |
BIter | begin () const |
Iterator for start of list. More... | |
BIter | end () const |
Iterator for end of list. More... | |
BIter | end (BIter &i) const |
Iterator for end of list. More... | |
void | next (BIter &i) const |
Iterator for next item in list. More... | |
void | prev (BIter &i) |
Iterator for previous item in list. More... | |
BIter | goTo (int pos) const |
Iterator for pos item in list. More... | |
int | position (BIter i) |
Postition in list item with iterator i. More... | |
unsigned int | number () const |
Number of items in list. More... | |
unsigned int | size () const |
Number of items in list. More... | |
int | isEnd (BIter &i) const |
True if iterator refers to last item. More... | |
BDictItem< Type > & | front () |
Get first item in list. More... | |
BDictItem< Type > & | rear () |
Get last item in list. More... | |
BDictItem< Type > & | get (BIter i) |
Get item specified by iterator in list. More... | |
const BDictItem< Type > & | get (BIter i) const |
Get item specified by iterator in list. More... | |
void | append (const BDictItem< Type > &item) |
Append item to list. More... | |
void | append (const BList< BDictItem< Type > > &l) |
Append list to list. More... | |
void | insertAfter (BIter &i, const BDictItem< Type > &item) |
Insert item after item. More... | |
void | deleteLast () |
Delete last item. More... | |
void | deleteFirst () |
Delete fisrt item. More... | |
void | push (const BDictItem< Type > &i) |
Push item onto list. More... | |
BDictItem< Type > | pop () |
Pop item from list deleteing item. More... | |
void | queueAdd (const BDictItem< Type > &i) |
Add item to end of list. More... | |
BDictItem< Type > | queueGet () |
Get item from front of list deleteing item. More... | |
int | has (const BDictItem< Type > &i) const |
Checks if the item is in the list. More... | |
void | swap (BIter i1, BIter i2) |
Swap two items in list. More... | |
void | sort () |
Sort list based on get(i) values. More... | |
void | sort (SortFunc func) |
Sort list based on Sort func. More... | |
BList< BDictItem< Type > > & | operator= (const BList< BDictItem< Type > > &l) |
BDictItem< Type > & | operator[] (int i) |
const BDictItem< Type > & | operator[] (int i) const |
BDictItem< Type > & | operator[] (BIter i) |
const BDictItem< Type > & | operator[] (const BIter &i) const |
BList< BDictItem< Type > > | operator+ (const BList< BDictItem< Type > > &l) const |
Private Member Functions | |
void | hashAdd (const BString &k, BIter iter) |
void | hashDelete (const BString &k, BIter iter) |
int | hashFind (const BString &k, BIter &iter) const |
Private Attributes | |
int | ohashSize |
BArray< BList< BIter > > | ohashLists |
Additional Inherited Members | |
![]() | |
virtual Node * | nodeGet (BIter i) |
virtual const Node * | nodeGet (BIter i) const |
virtual Node * | nodeCreate (const BDictItem< Type > &item) |
![]() | |
Node * | onodes |
unsigned int | olength |
Member Typedef Documentation
◆ iterator
Constructor & Destructor Documentation
◆ BDict() [1/2]
◆ BDict() [2/2]
Member Function Documentation
◆ append() [1/2]
◆ append() [2/2]
◆ clear()
template<class Type >
| virtual |
Clear the list.
Reimplemented from BList< BDictItem< Type > >.
◆ del() [1/2]
◆ del() [2/2]
Delete specified item.
Reimplemented from BList< BDictItem< Type > >.
◆ find()
◆ hashAdd()
◆ hashDelete()
◆ hashFind()
◆ hashPrint()
template<class Type >
void BDict< Type >::hashPrint | ( | ) |
◆ hasKey()
◆ insert()
template<class Type >
| virtual |
Insert item before item.
Reimplemented from BList< BDictItem< Type > >.
◆ key()
◆ operator+()
◆ operator=()
◆ operator[]() [1/4]
◆ operator[]() [2/4]
◆ operator[]() [3/4]
◆ operator[]() [4/4]
Member Data Documentation
◆ ohashLists
◆ ohashSize
template<class Type >
| private |
The documentation for this class was generated from the following file:
Generated by
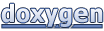