Beam-lib 2.15.1 |
#include <BTimeStamp.h>
Public Member Functions | |
BTimeStamp () | |
BTimeStamp (int year, int month=1, int day=1, int hour=0, int minute=0, int second=0, int microsecond=0) | |
BTimeStamp (const BString str) | |
~BTimeStamp () | |
void | clear () |
Clear the date/time. More... | |
void | setFirst () |
Set the first date available. More... | |
void | setLast () |
Set the last date available. More... | |
void | set (time_t time, int microSeconds) |
Set time using Unix time (seconds from 1970-01-01) More... | |
void | set (int year=0, int month=1, int day=1, int hour=0, int minute=0, int second=0, int microsecond=0) |
void | set (const BTimeStampMs &timeStamp) |
Set the timeStamp to given MS time stamp. More... | |
void | setYDay (int year=0, int yday=0, int hour=0, int minute=0, int second=0, int microsecond=0) |
void | setTime (int hour=0, int minute=0, int second=0, int microsecond=0) |
void | setNow () |
Set the timeStamp to now. More... | |
int | year () const |
int | yday () const |
int | month () const |
int | day () const |
int | hour () const |
int | minute () const |
int | second () const |
int | microSecond () const |
void | getDate (int &year, int &mon, int &day) const |
BString | getString (BString separator="T") const |
Get the time as an ISO date/time string. More... | |
BError | setString (const BString dateTime) |
Set the time from an ISO date/time. More... | |
BString | getStringNoMs (BString separator="T") const |
Get the time as an ISO date/time string without microseconds. More... | |
BString | getStringFormatted (BString format) const |
Gets the time in a string form as per the format. Format syntax as per strftime() More... | |
void | addMilliSeconds (int milliSeconds) |
Add the given number of milli seconds. This should be less that a year. More... | |
void | addMicroSeconds (int64_t microSeconds) |
Add the given number of micro seconds. This should be less that a year. More... | |
void | addSeconds (int seconds) |
Add the given number of seconds. This should be less that a year. More... | |
uint32_t | getYearSeconds () const |
Get number of seconds within the year. More... | |
uint64_t | getYearMicroSeconds () const |
Get number of micro seconds within the year. More... | |
int | isSet () const |
int | compare (const BTimeStamp &timeStamp) const |
Compare two dates. More... | |
operator BString () const | |
BTimeStamp & | operator= (const BTimeStampMs &timeStamp) |
int | operator== (const BTimeStamp &timeStamp) const |
int | operator!= (const BTimeStamp &timeStamp) const |
int | operator> (const BTimeStamp &timeStamp) const |
int | operator>= (const BTimeStamp &timeStamp) const |
int | operator< (const BTimeStamp &timeStamp) const |
int | operator<= (const BTimeStamp &timeStamp) const |
Static Public Member Functions | |
static int | isLeap (int year) |
static BInt64 | difference (BTimeStamp t2, BTimeStamp t1) |
Public Attributes | |
uint16_t | oyear |
Year (0 .. 65535) More... | |
uint16_t | oyday |
Day in year (0 .. 365) More... | |
uint8_t | ohour |
Hour (0 .. 23) More... | |
uint8_t | ominute |
Minute (0 .. 59) More... | |
uint8_t | osecond |
Second (0 .. 59) More... | |
uint8_t | ospare |
Padding. More... | |
uint32_t | omicroSecond |
MicroSecond (0 .. 999999) More... | |
Constructor & Destructor Documentation
◆ BTimeStamp() [1/3]
BTimeStamp::BTimeStamp | ( | ) |
◆ BTimeStamp() [2/3]
BTimeStamp::BTimeStamp | ( | int | year, |
int | month = 1 , | ||
int | day = 1 , | ||
int | hour = 0 , | ||
int | minute = 0 , | ||
int | second = 0 , | ||
int | microsecond = 0 | ||
) |
◆ BTimeStamp() [3/3]
BTimeStamp::BTimeStamp | ( | const BString | str | ) |
◆ ~BTimeStamp()
BTimeStamp::~BTimeStamp | ( | ) |
Member Function Documentation
◆ addMicroSeconds()
void BTimeStamp::addMicroSeconds | ( | int64_t | microSeconds | ) |
Add the given number of micro seconds. This should be less that a year.
◆ addMilliSeconds()
void BTimeStamp::addMilliSeconds | ( | int | milliSeconds | ) |
Add the given number of milli seconds. This should be less that a year.
◆ addSeconds()
void BTimeStamp::addSeconds | ( | int | seconds | ) |
Add the given number of seconds. This should be less that a year.
◆ clear()
void BTimeStamp::clear | ( | ) |
Clear the date/time.
◆ compare()
int BTimeStamp::compare | ( | const BTimeStamp & | timeStamp | ) | const |
Compare two dates.
◆ day()
int BTimeStamp::day | ( | ) | const |
◆ difference()
| static |
◆ getDate()
void BTimeStamp::getDate | ( | int & | year, |
int & | mon, | ||
int & | day | ||
) | const |
◆ getString()
Get the time as an ISO date/time string.
◆ getStringFormatted()
Gets the time in a string form as per the format. Format syntax as per strftime()
◆ getStringNoMs()
Get the time as an ISO date/time string without microseconds.
◆ getYearMicroSeconds()
uint64_t BTimeStamp::getYearMicroSeconds | ( | ) | const |
Get number of micro seconds within the year.
◆ getYearSeconds()
uint32_t BTimeStamp::getYearSeconds | ( | ) | const |
Get number of seconds within the year.
◆ hour()
int BTimeStamp::hour | ( | ) | const |
◆ isLeap()
| static |
◆ isSet()
| inline |
◆ microSecond()
int BTimeStamp::microSecond | ( | ) | const |
◆ minute()
int BTimeStamp::minute | ( | ) | const |
◆ month()
int BTimeStamp::month | ( | ) | const |
◆ operator BString()
| inline |
◆ operator!=()
| inline |
◆ operator<()
| inline |
◆ operator<=()
| inline |
◆ operator=()
| inline |
◆ operator==()
| inline |
◆ operator>()
| inline |
◆ operator>=()
| inline |
◆ second()
int BTimeStamp::second | ( | ) | const |
◆ set() [1/3]
void BTimeStamp::set | ( | time_t | time, |
int | microSeconds | ||
) |
Set time using Unix time (seconds from 1970-01-01)
◆ set() [2/3]
void BTimeStamp::set | ( | int | year = 0 , |
int | month = 1 , | ||
int | day = 1 , | ||
int | hour = 0 , | ||
int | minute = 0 , | ||
int | second = 0 , | ||
int | microsecond = 0 | ||
) |
◆ set() [3/3]
void BTimeStamp::set | ( | const BTimeStampMs & | timeStamp | ) |
Set the timeStamp to given MS time stamp.
◆ setFirst()
void BTimeStamp::setFirst | ( | ) |
Set the first date available.
◆ setLast()
void BTimeStamp::setLast | ( | ) |
Set the last date available.
◆ setNow()
void BTimeStamp::setNow | ( | ) |
Set the timeStamp to now.
◆ setString()
◆ setTime()
void BTimeStamp::setTime | ( | int | hour = 0 , |
int | minute = 0 , | ||
int | second = 0 , | ||
int | microsecond = 0 | ||
) |
◆ setYDay()
void BTimeStamp::setYDay | ( | int | year = 0 , |
int | yday = 0 , | ||
int | hour = 0 , | ||
int | minute = 0 , | ||
int | second = 0 , | ||
int | microsecond = 0 | ||
) |
◆ yday()
int BTimeStamp::yday | ( | ) | const |
◆ year()
int BTimeStamp::year | ( | ) | const |
Member Data Documentation
◆ ohour
uint8_t BTimeStamp::ohour |
Hour (0 .. 23)
◆ omicroSecond
uint32_t BTimeStamp::omicroSecond |
MicroSecond (0 .. 999999)
◆ ominute
uint8_t BTimeStamp::ominute |
Minute (0 .. 59)
◆ osecond
uint8_t BTimeStamp::osecond |
Second (0 .. 59)
◆ ospare
uint8_t BTimeStamp::ospare |
Padding.
◆ oyday
uint16_t BTimeStamp::oyday |
Day in year (0 .. 365)
◆ oyear
uint16_t BTimeStamp::oyear |
Year (0 .. 65535)
The documentation for this class was generated from the following files:
Generated by
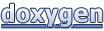