Beam-lib 2.15.1 |
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdarg.h>
#include <ctype.h>
#include <BString.h>
#include <BError.h>
#include <regex.h>
Macros | |
#define | STRIP 0x7f |
#define | MINUS '-' |
Functions | |
static int | gmatch (const char *s, const char *p) |
std::ostream & | operator<< (std::ostream &o, BString &s) |
std::istream & | operator>> (std::istream &i, BString &s) |
int | bstringListinList (BStringList &list, BString s) |
BString | blistToString (const BStringList &list) |
BStringList | bstringToList (BString str, int stripSpaces) |
BStringList | charToList (const char **str) |
BString | barrayToString (const BStringArray &list) |
BStringArray | bstringToArray (BString str, int stripSpaces) |
BStringArray | charToArray (const char **str) |
void | toBString (BString &v, BString &s) |
void | toBString (BStringList &v, BString &s) |
void | toBString (BInt32 &v, BString &s) |
void | toBString (BUInt32 &v, BString &s) |
void | toBString (BUInt64 &v, BString &s) |
void | toBString (BFloat64 &v, BString &s) |
void | fromBString (BString &s, BString &v) |
void | fromBString (BString &s, BStringList &v) |
void | fromBString (BString &s, BInt32 &v) |
void | fromBString (BString &s, BUInt32 &v) |
void | fromBString (BString &s, BUInt64 &v) |
void | fromBString (BString &s, BFloat64 &v) |
const char * | intToString (char *str, BUInt strLen, int value, int base) |
const char * | int64ToString (char *str, BUInt strLen, BInt64 value, int base) |
const char * | floatToString (char *str, BUInt strLen, BFloat32 f, BUInt precision) |
char * | bstrncpy (char *dest, const char *src, size_t n) |
char * | bstrtrim (char *str) |
Variables | |
static const BUInt8 | base64_decode_table [] |
Macro Definition Documentation
◆ MINUS
#define MINUS '-' |
◆ STRIP
#define STRIP 0x7f |
Function Documentation
◆ barrayToString()
BString barrayToString | ( | const BStringArray & | list | ) |
◆ blistToString()
BString blistToString | ( | const BStringList & | list | ) |
◆ bstringListinList()
int bstringListinList | ( | BStringList & | list, |
BString | s | ||
) |
◆ bstringToArray()
BStringArray bstringToArray | ( | BString | str, |
int | stripSpaces | ||
) |
◆ bstringToList()
BStringList bstringToList | ( | BString | str, |
int | stripSpaces | ||
) |
◆ bstrncpy()
char* bstrncpy | ( | char * | dest, |
const char * | src, | ||
size_t | n | ||
) |
◆ bstrtrim()
char* bstrtrim | ( | char * | str | ) |
◆ charToArray()
BStringArray charToArray | ( | const char ** | str | ) |
◆ charToList()
BStringList charToList | ( | const char ** | str | ) |
◆ floatToString()
◆ fromBString() [1/6]
◆ fromBString() [2/6]
void fromBString | ( | BString & | s, |
BStringList & | v | ||
) |
◆ fromBString() [3/6]
◆ fromBString() [4/6]
◆ fromBString() [5/6]
◆ fromBString() [6/6]
◆ gmatch()
| static |
◆ int64ToString()
◆ intToString()
const char* intToString | ( | char * | str, |
BUInt | strLen, | ||
int | value, | ||
int | base | ||
) |
◆ operator<<()
std::ostream& operator<< | ( | std::ostream & | o, |
BString & | s | ||
) |
◆ operator>>()
std::istream& operator>> | ( | std::istream & | i, |
BString & | s | ||
) |
◆ toBString() [1/6]
◆ toBString() [2/6]
void toBString | ( | BStringList & | v, |
BString & | s | ||
) |
◆ toBString() [3/6]
◆ toBString() [4/6]
◆ toBString() [5/6]
◆ toBString() [6/6]
Variable Documentation
◆ base64_decode_table
| static |
Initial value:
= {
66,66,66,66,66,66,66,66,66,66,64,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,62,66,66,66,63,52,53,
54,55,56,57,58,59,60,61,66,66,66,65,66,66,66, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,66,66,66,66,66,66,26,27,28,
29,30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49,50,51,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
66,66,66,66,66,66
}
Generated by
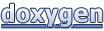