Account
LibTmsApi 2.3.0 |
This interface provides functions to control, test and get statistics from the TMS as a whole. More...
#include <TmsC.h>
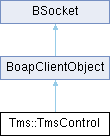
Public Member Functions | |
TmsControl (BString name="") | |
BError | getVersion (BString &version) |
Gets the software version. | |
BError | setProcessPriority (BUInt32 priority) |
Sets the priority of the process servicing this service. | |
BError | init () |
Initialises the system including resetting all of the PUPE engines firmware. The call will return an error object indicating success or an error condition as appropriate. | |
BError | configure (ConfigInfo configInfo) |
Configure the system for use. This includes mapping the individual physical PickUp channels to logical pickup channels. | |
BError | getConfiguration (ConfigInfo &configInfo) |
Get the current configuration. | |
BError | setControlInfo (CycleParam params) |
Sets the control information for the cycle type given. The parameters for the processing cycle are passed, this includes the Phase and State table information. The call will return an error object indicating success or an error. | |
BError | getControlInfo (BString cycleType, BUInt32 ring, BUInt32 puChannel, CycleParam ¶ms) |
Gets the control information for the cycle type and puChannel number given. The call will return an error object indicating success or an error. | |
BError | delControlInfo (BString cycleType, BUInt32 ring, BUInt32 puChannel) |
Deletes the control information for the cycle type and puChannel number given. The call will return an error object indicating success or an error. | |
BError | getControlList (BList< CycleParamItem > &itemList) |
Gets the list of Cycle Parameters present in the system. | |
BError | setNextCycle (BUInt32 cycleNumber, BString cycleType) |
Sets the cycle number and type for the next processing cycle. The call will return an error object indicating success or an error condition as appropriate. This should be called at least 10ms before the next CYCLE_START event. | |
BError | test (BList< BError > &errors) |
Performs a basic test of the system returning a list of errors. The call will return an error object indicating success or an error condition as appropriate. | |
BError | getStatus (BList< NameValue > &statusList) |
Returns the current status of the system. This information includes the number of Pick-Up's present and their individual status. | |
BError | getStatistics (BList< NameValue > &statsList) |
Returns a list of the statistic values as name/value pairs. The call will return an error object indicating success or an error condition as appropriate. | |
BError | getPuChannel (BUInt32 puChannel, PuChannel &puPhysChannel) |
Returns the physical module/Pupe/Channel number given a logical PickUp id. This can be used so that the individual PickUps test functions can be accessed etc. | |
BError | setSimulation (Simulation simulation) |
Sets overall simulation modes. | |
BError | getSimulation (Simulation &simulation) |
Gets current simulation modes. | |
BError | setTestMode (PuChannel puPhysChannel, BUInt32 testOutput, BUInt32 timingDisableMask) |
The signal source for the digital test output connector. 0: None, 1: FrefLocal. The timingDisableMask bit mask defines which of the timing inputs should be disabled. If a timing input is disabled it can be still operated by software command. | |
BError | setTimingSignals (PuChannel puPhysChannel, BUInt32 timingSignals) |
This function sets the given timing signals to the values as defined in the timingSignals bit array. | |
BError | captureDiagnostics (PuChannel puPhysChannel, TestCaptureInfo captureInfo, BArray< BUInt64 > &data) |
This function will capture the diagnostics. | |
BError | setTestData (PuChannel puPhysChannel, BInt32 on, BArray< BUInt32 > data) |
This function will set a PU channel to sample data from memory rather than the ADC's. | |
BError | setPupeConfig (PuChannel puPhysChannel, PupeConfig pupeConfig) |
Sets special PUPE configuration for test purposes. | |
BError | getPupeConfig (PuChannel puPhysChannel, PupeConfig &pupeConfig) |
Gets special PUPE configuration for test purposes. | |
BError | puServerStarted (BUInt32 number) |
A TmsPuServer has started. | |
![]() | |
BoapClientObject (BString name="") | |
virtual | ~BoapClientObject () |
BError | connectService (BString name) |
Connects to the named service. | |
BError | disconnectService () |
Disconnects from the named service. | |
BString | getServiceName () |
Get the name of the service. | |
BError | ping (BUInt32 &apiVersion) |
Pings the connection and finds the remotes version number. | |
BError | setConnectionPriority (BoapPriority priority) |
Sets the connection priority. | |
void | setMaxLength (BUInt32 maxLength) |
Sets the maximum packet length. | |
void | setTimeout (int timeout) |
Sets the timeout in micro seconds. -1 is wait indefinitely. | |
BoapClientObject (BString name) | |
BError | connectService (BString name) |
![]() | |
BSocket () | |
BSocket (int fd) | |
BSocket (NType type) | |
BSocket (int domain, int type, int protocol) | |
~BSocket () | |
BError | init (int domain, int type, int protocol) |
BError | init (NType type) |
void | setFd (int fd) |
int | getFd () |
BError | bind (const BSocketAddress &add) |
BError | connect (const BSocketAddress &add) |
BError | shutdown (int how) |
BError | close () |
BError | listen (int backlog=5) |
BError | accept (int &fd) |
BError | accept (int &fd, BSocketAddress &address) |
BError | send (const void *buf, BSize nbytes, BSize &nbytesSent, int flags=0) |
BError | sendTo (const BSocketAddress &address, const void *buf, BSize nbytes, BSize &nbytesSent, int flags=0) |
BError | recv (void *buf, BSize maxbytes, BSize &nbytesRecv, int flags=0) |
BError | recvFrom (BSocketAddress &address, void *buf, BSize maxbytes, BSize &nbytesRecv, int flags=0) |
BError | recvWithTimeout (void *buf, BSize maxbytes, BSize &nbytesRecv, int timeout, int flags=0) |
BError | recvFromWithTimeout (BSocketAddress &address, void *buf, BSize maxbytes, BSize &nbytesRecv, int timeout, int flags=0) |
BError | setSockOpt (int level, int optname, void *optval, unsigned int optlen) |
BError | getSockOpt (int level, int optname, void *optval, unsigned int *optlen) |
BError | setReuseAddress (int on) |
BError | setBroadCast (int on) |
BError | setPriority (Priority priority) |
BError | getMTU (uint32_t &mtu) |
BError | getAddress (BSocketAddress &address) |
Detailed Description
This interface provides functions to control, test and get statistics from the TMS as a whole.
Constructor & Destructor Documentation
◆ TmsControl()
Tms::TmsControl::TmsControl | ( | BString | name = "" | ) |
Member Function Documentation
◆ captureDiagnostics()
BError Tms::TmsControl::captureDiagnostics | ( | PuChannel | puPhysChannel, |
TestCaptureInfo | captureInfo, | ||
BArray< BUInt64 > & | data | ||
) |
This function will capture the diagnostics.
◆ configure()
BError Tms::TmsControl::configure | ( | ConfigInfo | configInfo | ) |
Configure the system for use. This includes mapping the individual physical PickUp channels to logical pickup channels.
- Parameters
-
configInfo The channel mapping table.
This function configures the logical to physical channel mapping table.
◆ delControlInfo()
Deletes the control information for the cycle type and puChannel number given. The call will return an error object indicating success or an error.
- Parameters
-
cycleType This string defines the cycle type to delete from the database. puChannel This defines the specific channel to delete the information for. 0 means all channels.
This function will delete a set of Cycle parameters from the TMS's Cycle parameter database.
◆ getConfiguration()
BError Tms::TmsControl::getConfiguration | ( | ConfigInfo & | configInfo | ) |
Get the current configuration.
- Parameters
-
configInfo The channel mapping table that is filled in with the current curent channel mapping.
This function reads the current logical to physical channel mapping table.
◆ getControlInfo()
BError Tms::TmsControl::getControlInfo | ( | BString | cycleType, |
BUInt32 | ring, | ||
BUInt32 | puChannel, | ||
CycleParam & | params | ||
) |
Gets the control information for the cycle type and puChannel number given. The call will return an error object indicating success or an error.
- Parameters
-
cycleType This string defines the cycle type for which to get the information. puChannel This defines the channel to get the information for. 0 means all channels. params The resuting cycle parameters are placed in this object.
This function reads back the set of Cycle parameters for the given cycle type and channel number. Normall the same cycle parameters are used for all PUPE engines. In this case setting the puChannel to 0 reads the Cycle Parameters that are being used on all channels. If a specific channel has other parameters the puChannel variable can be set to the appropriate channel number to get its particular settings.
◆ getControlList()
BError Tms::TmsControl::getControlList | ( | BList< CycleParamItem > & | itemList | ) |
Gets the list of Cycle Parameters present in the system.
- Parameters
-
itemList The list of CycleType information is returned.
This function will return a list of entries describing the Cycle Paramter sets present in the TMS database.
◆ getPuChannel()
Returns the physical module/Pupe/Channel number given a logical PickUp id. This can be used so that the individual PickUps test functions can be accessed etc.
- Parameters
-
puChannel The logical channel number. puPhysChannel The physical channel identifier is returned in this variable.
This function is given a logical pick-up channel number. It will return the physical module, pupe number and pupe channle that has been allocated to this channel.
◆ getPupeConfig()
BError Tms::TmsControl::getPupeConfig | ( | PuChannel | puPhysChannel, |
PupeConfig & | pupeConfig | ||
) |
Gets special PUPE configuration for test purposes.
- Parameters
-
puPhysChannel The physical channel identifier. pupeConfig The returned configuration parameters.
This function returns the current configuration of the given channel.
◆ getSimulation()
BError Tms::TmsControl::getSimulation | ( | Simulation & | simulation | ) |
Gets current simulation modes.
◆ getStatistics()
Returns a list of the statistic values as name/value pairs. The call will return an error object indicating success or an error condition as appropriate.
- Parameters
-
statsList The statistics list is placed in this list object.
This function gets the statistics values from the TMS system. It returns a list of name/value pairs.
◆ getStatus()
Returns the current status of the system. This information includes the number of Pick-Up's present and their individual status.
- Parameters
-
statusList The list of status items is placed in this list object.
This function gets the status of the TMS system. It returns a list of name/value pairs.
◆ getVersion()
Gets the software version.
- Parameters
-
version A string variable filled in with the version number string.
◆ init()
BError Tms::TmsControl::init | ( | ) |
Initialises the system including resetting all of the PUPE engines firmware. The call will return an error object indicating success or an error condition as appropriate.
This function restarts the TMS system. It re-initialises each of the TmsPuServer processes running on the Module Controllers and reboots each of the PUPE boards from scratch loading the current FPGA firmware. All errors and statistics values are reset.
◆ puServerStarted()
A TmsPuServer has started.
- Parameters
-
number The number of the PuServer started.
This is an internal function called by the TmsPuServer processes to indicate to the TmsServer that they have just started running and are present in the system. The TmsServer will initialise the appropriate tmsPuServer program and its individual PUPE engines on receipt of this call.
◆ setControlInfo()
BError Tms::TmsControl::setControlInfo | ( | CycleParam | params | ) |
Sets the control information for the cycle type given. The parameters for the processing cycle are passed, this includes the Phase and State table information. The call will return an error object indicating success or an error.
- Parameters
-
params Cycle information parameters (state/phase table information).
This function over-writes or adds an entry in the Cycle Parameter database. The Cycle Parameters define the setting for each processing cycle including the state and phase tables for the PUPE FPGA engines.
◆ setNextCycle()
Sets the cycle number and type for the next processing cycle. The call will return an error object indicating success or an error condition as appropriate. This should be called at least 10ms before the next CYCLE_START event.
- Parameters
-
cycleNumber This is the next cycle number. This should be an incrementing 32bit unsigned value. cycleType This is a string defining the cycle type for the next cycle.
This call configures the TMS system for the next processing cycle. It defines the cycle number that will be used to tag data captured during the cycle and it defines the type of machine cycle. The cycleType is used to lookup the appropriate state/phase table information to use in the FPGA's. The call should be made at least 10ms before the CYCLE_START event for the cycle it refers to. This gives time for the FPGA's to be loaded with the appropriate state/phase table information. As the function is time critical, the communications channel should be set to a high priority using the setPriority() call and the processing threads priority should be set to high using the setProcessPriority() call. The call will return the error: "ErrorCycleNumber", "The next cycle has already started" if the call has not completed before the CYCLE_START event. All client data reads, for this cycle, will also return this error message.
◆ setProcessPriority()
Sets the priority of the process servicing this service.
- Parameters
-
priority This is the priority of the process. It can be set to one of: PriorityLow, PriorityNormal, PriorityHigh.
◆ setPupeConfig()
BError Tms::TmsControl::setPupeConfig | ( | PuChannel | puPhysChannel, |
PupeConfig | pupeConfig | ||
) |
Sets special PUPE configuration for test purposes.
- Parameters
-
puPhysChannel The physical channel identifier. pupeConfig The configuration parameters to use.
This functions sets up some special configuration parameters for the PUPE channel. It is used mainly for diagnostics and test purposes. The main settings it can affect are: The ADC Clock sources PLL synchronisation, internal timing for the digtital timing signals and the enabling/dissabling of the BLR algorithem.
◆ setSimulation()
BError Tms::TmsControl::setSimulation | ( | Simulation | simulation | ) |
Sets overall simulation modes.
◆ setTestData()
This function will set a PU channel to sample data from memory rather than the ADC's.
- Parameters
-
puPhysChannel The physical channel identifier. on Boolean to enable the internal data source. 0 is off, 1 is on. data The array of 32bit data values to use as the FREF,Sigma,DeltaX and DeltaY test signal.
This call loads the PUPE systems test data SDRAM with the data passed in the data array. It then sets up the individual channel to sources its FREF, Sigma, DeltaX and DelatY signals from the test SDRAM. The data source should have a multiple of 2 samples. The "on" parameter is used to enable or disable the individual channels inputs from this test data SDRAM.
◆ setTestMode()
BError Tms::TmsControl::setTestMode | ( | PuChannel | puPhysChannel, |
BUInt32 | testOutput, | ||
BUInt32 | timingDisableMask | ||
) |
The signal source for the digital test output connector. 0: None, 1: FrefLocal. The timingDisableMask bit mask defines which of the timing inputs should be disabled. If a timing input is disabled it can be still operated by software command.
- Parameters
-
puPhysChannel The physical channel identifier. testOutput The signal to output on the test output. 0 is FREF any other value is undefined at the moment. timingDisableMask This 8 bit mask defines which of the timing input signals are disabled.
This function sets up a particular pick-up channel's digital test output source and allows the channels input timing signals to be set to a software driven mode rather than taken from the hardware timing inputs. The timing mask bits are: 7 - FREF, 6 - HCHANGE, 5 - INJECTION, 4 - CAL_STOP, 3 - CAL_START, 2 - CYCLE_STOP, 1 - CYCLE_START, 0 - SYSCLOCK
◆ setTimingSignals()
This function sets the given timing signals to the values as defined in the timingSignals bit array.
- Parameters
-
puPhysChannel The physical channel identifier. timingSignals The 8 bit mask defining the state of the software driven timing signals.
If the setTestMode() function had been used to "enable" particular timing signals to be driven by software, then this function can be used to set/reset particular timing signals for the pick-up channel given. The timing signals bits are: 7 - FREF, 6 - HCHANGE, 5 - INJECTION, 4 - CAL_STOP, 3 - CAL_START, 2 - CYCLE_STOP, 1 - CYCLE_START, 0 - SYSCLOCK
◆ test()
Performs a basic test of the system returning a list of errors. The call will return an error object indicating success or an error condition as appropriate.
- Parameters
-
errors The list of errors is placed in this list object.
This function will perform a test of the TMS system. It will report each test performed and the status of the test in the BError object. A status value of 0 indicates all was Ok, any other value is an error where the number indicates the error. A string gives the test name and the Ok or error condition as a string.
The documentation for this class was generated from the following files:
Generated by