Account
LibTmsApi 2.3.0 |
BEntryFile Class Reference
File of Entries. More...
#include <BEntry.h>
Inheritance diagram for BEntryFile:
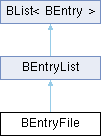
Public Member Functions | |
BEntryFile () | |
BEntryFile (BString filename) | |
Opens entryfile. | |
~BEntryFile () | |
int | open (BString filename) |
Opens entryfile. | |
int | read () |
Reads entry file and builds list. | |
int | write () |
Writes list to entryfile. | |
int | writeList (BEntryList &l) |
Writes specified list to file. | |
void | clear () |
Clears current list. | |
BString | filename () |
Returns the filename. | |
![]() | |
BEntryList () | |
int | isSet (BString name) |
1 if name is in list and value is set | |
BEntry * | find (BString name) |
Returns entry if name is found otherwise NULL. | |
BString | findValue (BString name) |
Returns value of name. Returns "" if name not found. | |
int | setValue (BString name, BString value) |
Set the value of name. Returns 0 if name not found. | |
int | setValueRaw (BString name, BString value) |
Raw setting of value without looking up existing entry. | |
void | deleteEntry (BString name) |
Deletes the entry. | |
void | print () |
Print list. | |
BString | getString () |
Return list as string. Each Entry padded and on a new line. | |
void | insert (BIter &i, const BEntry &item) |
void | del (BIter &i) |
Delete specified item. | |
void | clear () |
Clear the list. | |
BEntryList & | operator= (const BEntryList &l) |
![]() | |
BList () | |
BList (const BList< BEntry > &l) | |
virtual | ~BList () |
void | start (BIter &i) const |
Iterator to start of list. | |
BIter | begin () const |
Iterator for start of list. | |
BIter | end () const |
Iterator for end of list. | |
BIter | end (BIter &i) const |
Iterator for end of list. | |
void | next (BIter &i) const |
Iterator for next item in list. | |
void | prev (BIter &i) |
Iterator for previous item in list. | |
BIter | goTo (int pos) const |
Iterator for pos item in list. | |
int | position (BIter i) |
Postition in list item with iterator i. | |
unsigned int | number () const |
Number of items in list. | |
unsigned int | size () const |
Number of items in list. | |
int | isEnd (BIter &i) const |
True if iterator refers to last item. | |
BEntry & | front () |
Get first item in list. | |
BEntry & | rear () |
Get last item in list. | |
BEntry & | get (BIter i) |
Get item specified by iterator in list. | |
const BEntry & | get (BIter i) const |
Get item specified by iterator in list. | |
void | append (const BEntry &item) |
Append item to list. | |
void | append (const BList< BEntry > &l) |
Append list to list. | |
virtual void | insert (BIter &i, const BEntry &item) |
Insert item before item. | |
void | insertAfter (BIter &i, const BEntry &item) |
Insert item after item. | |
virtual void | clear () |
Clear the list. | |
virtual void | del (BIter &i) |
Delete specified item. | |
void | deleteLast () |
Delete last item. | |
void | deleteFirst () |
Delete fisrt item. | |
void | push (const BEntry &i) |
Push item onto list. | |
BEntry | pop () |
Pop item from list deleteing item. | |
void | queueAdd (const BEntry &i) |
Add item to end of list. | |
BEntry | queueGet () |
Get item from front of list deleteing item. | |
int | has (const BEntry &i) const |
Checks if the item is in the list. | |
void | swap (BIter i1, BIter i2) |
Swap two items in list. | |
void | sort () |
Sort list based on get(i) values. | |
void | sort (SortFunc func) |
Sort list based on Sort func. | |
BList< BEntry > & | operator= (const BList< BEntry > &l) |
BEntry & | operator[] (int i) |
const BEntry & | operator[] (int i) const |
BEntry & | operator[] (BIter i) |
const BEntry & | operator[] (const BIter &i) const |
BList< BEntry > | operator+ (const BList< BEntry > &l) const |
Private Attributes | |
BString | ofilename |
BString | ocomments |
Additional Inherited Members | |
![]() | |
typedef int(* | SortFunc) (BEntry &a, BEntry &b) |
Prototype for sorting function. | |
![]() | |
virtual Node * | nodeGet (BIter i) |
virtual const Node * | nodeGet (BIter i) const |
virtual Node * | nodeCreate (const BEntry &item) |
![]() | |
Node * | onodes |
unsigned int | olength |
Detailed Description
File of Entries.
Constructor & Destructor Documentation
◆ BEntryFile() [1/2]
BEntryFile::BEntryFile | ( | ) |
◆ BEntryFile() [2/2]
BEntryFile::BEntryFile | ( | BString | filename | ) |
Opens entryfile.
◆ ~BEntryFile()
BEntryFile::~BEntryFile | ( | ) |
Member Function Documentation
◆ clear()
| virtual |
Clears current list.
Reimplemented from BList< BEntry >.
◆ filename()
BString BEntryFile::filename | ( | ) |
Returns the filename.
◆ open()
int BEntryFile::open | ( | BString | filename | ) |
Opens entryfile.
◆ read()
int BEntryFile::read | ( | ) |
Reads entry file and builds list.
◆ write()
int BEntryFile::write | ( | ) |
Writes list to entryfile.
◆ writeList()
int BEntryFile::writeList | ( | BEntryList & | l | ) |
Writes specified list to file.
Member Data Documentation
◆ ocomments
| private |
◆ ofilename
| private |
The documentation for this class was generated from the following files:
- /src/cern/tms/tms/beam/libBeam/BEntry.h
- /src/cern/tms/tms/beam/libBeam/BEntry.cpp
Generated by